User guide
Input files
Configuration file
Configuration name |
Data type |
Description |
---|---|---|
NB_PHOTONS |
int |
The number of photons used in the simulation |
MAXIMUM_DEPTH |
int |
The maximum number of times that the light bounces
in the scene
|
SCALE_FACTOR |
int |
The size of geometries. The vertices of geometries
is recalculated by dividing their coordinates by
this value
|
T_MIN |
int |
The minimum distance between the point of
intersection and the origin of the light ray
|
NB_THREAD |
int |
The number of threads on the CPU used to calculate in
parallel. This value is between 0 and the number of
cores of your CPU.
|
BACKFACE_CULLING |
yes/no |
Define which mode of intersection is chosen: intersect
only with the front face (yes) or intersect with both
faces (no)
|
BASE_SPECTRAL_RANGE |
int int |
The base spectral range which includes all the other
spectral ranges. The first value is the start of band
and the second is the end of band. Ex: 100 200
|
DIVIDED_SPECTRAL_RANGE |
int [int int] |
The list of spectral ranges divided from the base
spectral range. The first value is the number of
divided parts, the value start and end of band is
continue right after. These bands have to be smaller
than the base spectral range. Ex: 2 100 150 150 200
|
$NB_PHOTONS 1000000000
$MAXIMUM_DEPTH 50
$SCALE_FACTOR 1
$T_MIN 0.1
$NB_THREAD 8
$BACKFACE_CULLING yes
$BASE_SPECTRAL_RANGE 400 800
$DIVIDED_SPECTRAL_RANGE 2 600 655 655 665
Room file
.rad
is supported by our outil..rad
, there are two steps:void material_type material_name
0
0
material_data
material_name geometry_type object_name
0
0
geometry_data
geometry_type
and material_type
, we will have the different ways to define the geometry_data
and material_data
polygon
and the material type metal
void metal Sol
0
0
5
0.37047683515999996 0.37047683515999996 0.37047683515999996 0.100 0
Sol polygon dallage
0
0
12
0.0 0.0 0
2400.0 0.0 0
2400.0 1840.0 0
0.0 1840.0 0
.rad
can be found in this file refman.pdfOptical property files
The files containing the optical properties are saved in this structure of folder:
Materiau |
Valeur estimee visuellement |
---|---|
Aquanappe |
0.1 |
CentrePlafond |
0.5 |
CorniereAlu |
0.3 |
PiedsTablette |
0.3 |
MiroirCaissonLampes |
0.4 |
lambda |
moy |
---|---|
300 |
0.126 |
301 |
0.135 |
302 |
0.145 |
… |
… |
Captor file
X |
Y |
Z |
rayon_capteur |
Xnorm |
Ynorm |
Znorm |
---|---|---|---|---|---|---|
110 |
930 |
1000 |
10 |
0 |
0 |
1 |
210 |
930 |
1000 |
10 |
0 |
0 |
1 |
310 |
930 |
1000 |
10 |
0 |
0 |
1 |
… |
… |
… |
… |
… |
… |
… |
Plant file
Spectral heterogeneity file
wavelength(nm) |
measured PPFD (umol m-2 s-1 nm-1) |
---|---|
401 |
0.0555 |
403 |
0.086 |
405 |
0.14 |
… |
… |
Calibration points file
X |
Y |
Z |
Nmes_start1_end1 |
Nmes_start2_end2 |
… |
Nmes_startn_endn |
---|---|---|---|---|---|---|
610 |
1330 |
1400 |
3.91336 |
19.6182 |
… |
value_1 |
710 |
1330 |
1400 |
4.17343 |
20.8869 |
… |
value_2 |
1210 |
1330 |
1400 |
3.80179 |
18.8231 |
… |
value_3 |
… |
… |
… |
… |
… |
… |
… |
Run a simple simulation
There are the basic steps to run a simple light simulation with this tool
Setup input files
Create a configuration file (simulation.ini)
$NB_PHOTONS 1000000000
$MAXIMUM_DEPTH 50
$SCALE_FACTOR 1
$T_MIN 0.1
$NB_THREAD 8
$BACKFACE_CULLING yes
$BASE_SPECTRAL_RANGE 400 800
$DIVIDED_SPECTRAL_RANGE 2 600 655 655 665
Create a room file (testChamber.rad) with only one light
void light lum400
0
0
3
0.4 0.4 0.4
lum400 cylinder lamp
0
0
7
715.62 1670.0 2105.0
743.193 1670.0 2105.0
0.1
Create a captor file (captors_expe1.csv) with only one captor
X,Y,Z,rayon_capteur,Xnorm,Ynorm,Znorm
110,930,1000,10,0,0,1
The calibration points file and spectral heterogeneity file are optional
Write the core program
Create a python file (main.py) which contains the core program
from photonmap.Simulator import *
if __name__ == "__main__":
simulator = Simulator()
simulator.readConfiguration("simulation.ini")
simulator.addEnvFromFile("testChamber.rad", "./PO")
simulator.addVirtualDiskCaptorsFromFile("captors_expe1.csv")
res = simulator.run()
res.writeResults() #write results to a file
Run and results
conda activate env_name
python main.py
id |
N_sim_600_655 |
N_sim_655_665 |
---|---|---|
0 |
13635 |
13966 |
Calibrate the results
calibrateResults
.calibrated_res = simulator.calibrateResults("spectrum/chambre1_spectrum", "points_calibration.csv")
calibrated_res.writeResults() #write results to a file
Linear Regression
to calculate the coefficients to convertir the results of simulation to irradiance (a unit used to measure the power of energy).Visualize the room
To visualize the room, after defining the input files, we use a function named visualiserSimulationScene
. Here is the complete code for this program:
from photonmap.Simulator import *
if __name__ == "__main__":
simulator = Simulator()
simulator.readConfiguration("simulation.ini")
simulator.addEnvFromFile("testChamber.rad", "./PO")
simulator.addVirtualDiskCaptorsFromFile("captors_expe1.csv")
simulator.visualiserSimulationScene("ipython")
To obtain the 3D scene, we have to run this program through `ipython`
.
ipython
%gui qt5
run main.py
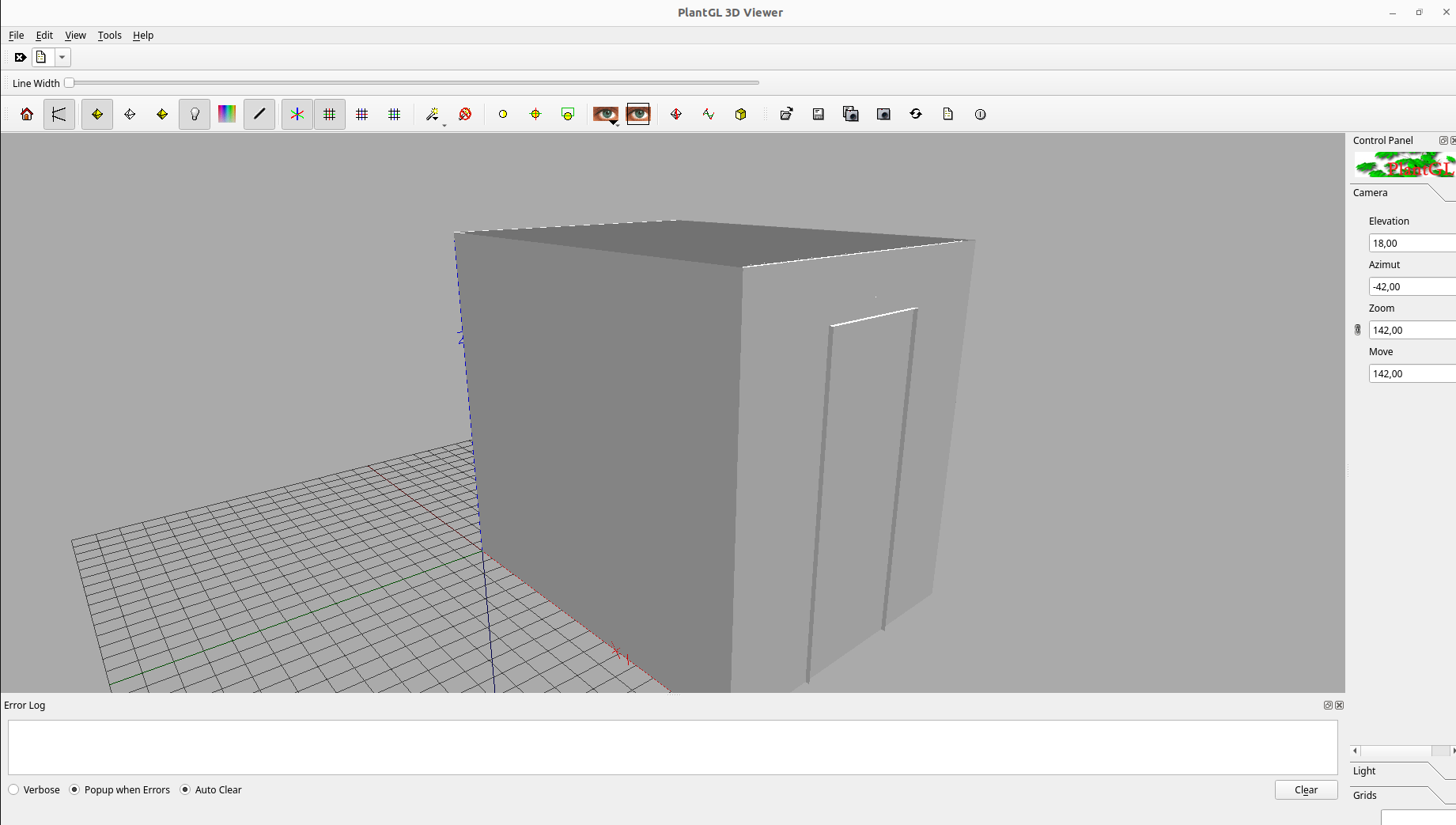
Test value Tmin
Tmin
is too small.Tmin
. The result of this function is a graph showing the change in the number of photons after testing different values of Tmin
.from photonmap.Simulator import *
if __name__ == "__main__":
simulator = Simulator()
simulator.readConfiguration("simulation.ini")
simulator.addEnvFromFile("testChamber.rad", "./PO")
simulator.addVirtualDiskCaptorsFromFile("captors_expe1.csv")
simulator.test_t_min(int(1e6), 1e-6, 10, True)
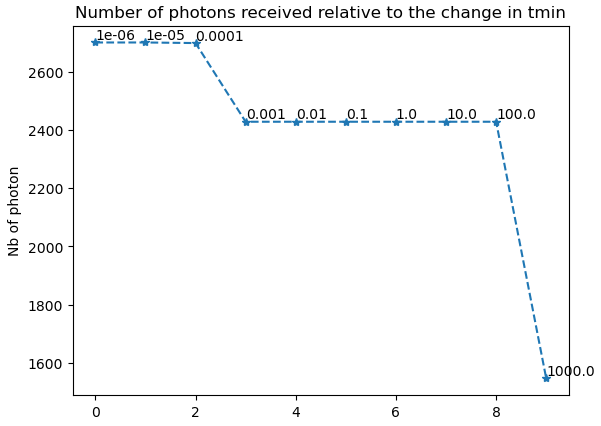